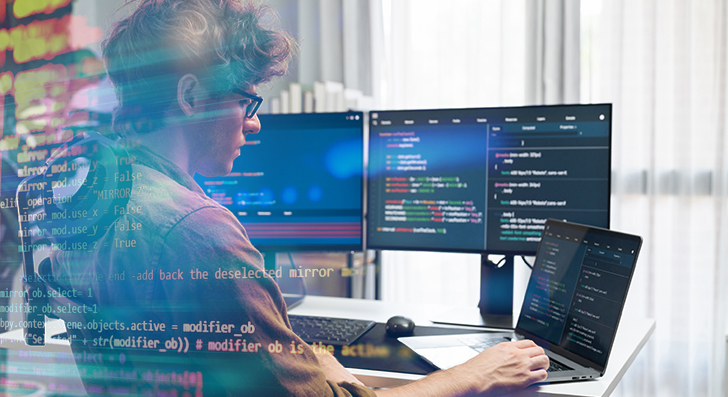
Scalability signifies your software can cope with progress—much more users, additional knowledge, and even more visitors—without breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your prepare from the beginning. Many apps are unsuccessful whenever they grow rapidly because the initial design can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your procedure will behave under pressure.
Start out by creating your architecture to get adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day one particular. Will it have to have to handle a million consumers or merely 100? Pick the right kind—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, Even when you don’t require them however.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only works under present conditions. Consider what would occur In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style and design styles that support scaling, like message queues or event-pushed units. These assistance your application tackle extra requests without the need of getting overloaded.
Once you Construct with scalability in mind, you are not just making ready for achievement—you are decreasing long term headaches. A perfectly-prepared procedure is simpler to keep up, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the appropriate databases can be a important part of making scalable programs. Not all databases are constructed the same, and utilizing the Improper one can sluggish you down or maybe result in failures as your application grows.
Start off by knowing your data. Can it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to deal with extra targeted visitors and knowledge.
If your knowledge is more versatile—like person activity logs, product or service catalogs, or documents—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your study and produce styles. Have you been doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a large generate load? Consider databases that could tackle high produce throughput, or even occasion-based mostly facts storage units like Apache Kafka (for short-term info streams).
It’s also sensible to Assume in advance. You might not require State-of-the-art scaling features now, but choosing a database that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your knowledge according to your accessibility designs. And constantly keep an eye on databases effectiveness while you increase.
Briefly, the appropriate databases is dependent upon your application’s composition, velocity requires, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve plenty of problems afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, each individual compact hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting cleanse, straightforward code. Steer clear of repeating logic and take away nearly anything needless. Don’t choose the most complicated solution if a straightforward a single functions. Keep the features short, centered, and easy to check. Use profiling equipment to locate bottlenecks—sites where by your code can take also long to run or utilizes an excessive amount memory.
Up coming, look at your database queries. These often sluggish things down a lot more than the code by itself. Make sure Every single query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And click here keep away from doing too many joins, In particular across huge tables.
For those who discover the exact same data getting asked for again and again, use caching. Retailer the final results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your app more productive.
Make sure to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could crash when they have to handle 1 million.
In brief, scalable apps are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application stay easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with additional people plus more targeted visitors. If all the things goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching can be found in. Both of these equipment aid keep your app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the function, the load balancer routes users to different servers dependant on availability. What this means is no solitary server will get overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same info all over again—like an item web page or simply a profile—you don’t ought to fetch it in the databases each and every time. You can provide it in the cache.
There's two frequent types of caching:
1. Server-facet caching (like Redis or Memcached) suppliers info in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files close to the person.
Caching minimizes databases load, improves pace, and will make your app much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does alter.
Briefly, load balancing and caching are simple but strong applications. With each other, they assist your application deal with extra end users, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application mature effortlessly. That’s wherever cloud platforms and containers come in. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to invest in components or guess future capacity. When targeted traffic boosts, you may increase extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You may center on setting up your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be easy to maneuver your application among environments, from your laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent elements of your application into companies. You are able to update or scale pieces independently, that's great for functionality and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy easily, and Get well quickly when troubles happen. If you need your app to improve with out boundaries, begin employing these tools early. They preserve time, cut down danger, and make it easier to stay focused on making, not correcting.
Check Anything
If you don’t check your software, you received’t know when issues go Mistaken. Checking assists the thing is how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of making scalable systems.
Begin by tracking standard metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this info.
Don’t just keep an eye on your servers—watch your application too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes earlier mentioned a limit or even a support goes down, you ought to get notified instantly. This assists you fix issues speedy, generally in advance of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again just before it leads to serious hurt.
As your app grows, traffic and facts boost. With out checking, you’ll overlook indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works perfectly, even under pressure.
Closing Thoughts
Scalability isn’t only for big corporations. Even little applications need a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking stressed. Begin smaller, think huge, and Make smart.